In "Array"
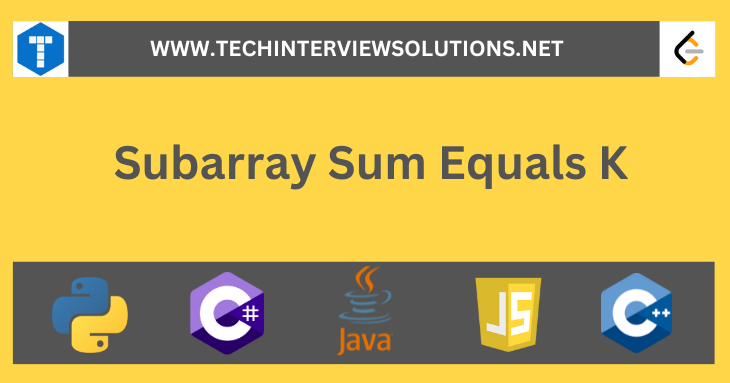
Subarray Sum Equals K
Given an array of integers nums and an integer k, return the total number of subarrays whose sum equals...
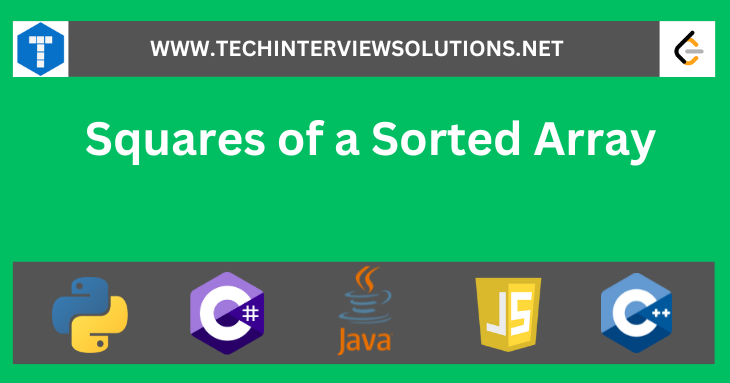
Squares of a Sorted Array
Given an integer array nums sorted in non-decreasing order, return an array of the squares of each number sorted in non-decreasing order....
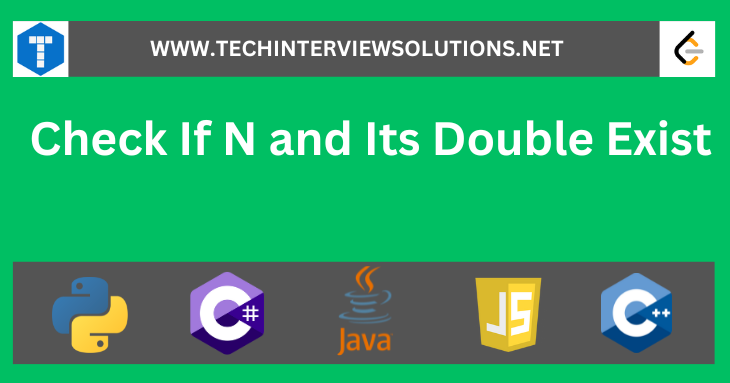
Check If N and Its Double Exist
Given an array arr of integers, check if there exist two indices i and j such that :
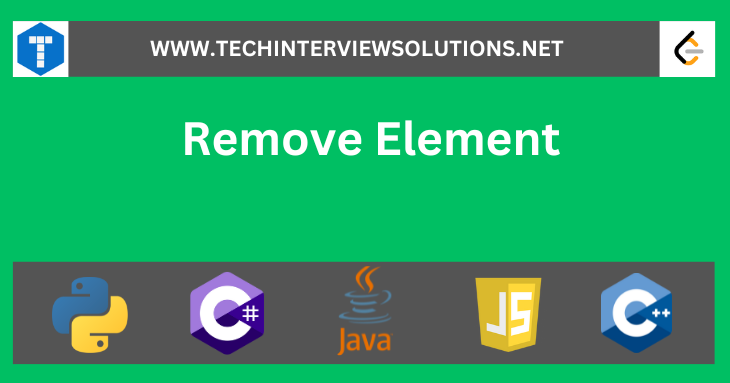
Remove Element
Given an integer array nums and an integer val, remove all occurrences of val in nums in-place. The relative order of the...
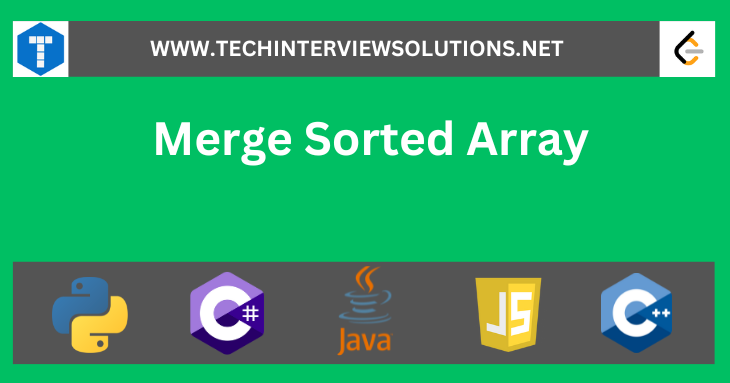
Merge Sorted Array
You are given two integer arrays nums1 and nums2, sorted in non-decreasing order, and two integers m and n, representing the number...
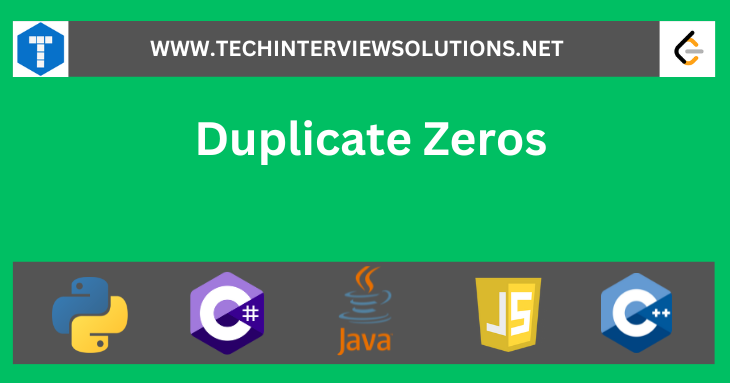
Duplicate Zeros
Given a fixed-length integer array arr, duplicate each occurrence of zero, shifting the remaining elements to...
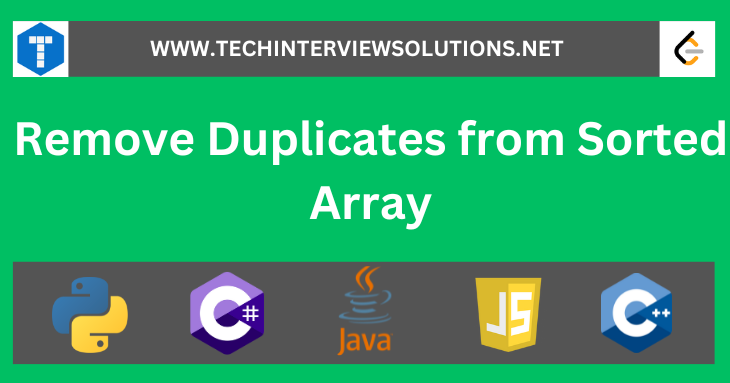
Remove Duplicates from Sorted Array
Given an integer array nums sorted in non-decreasing order, remove the duplicates in-place such that each unique element appears only once....
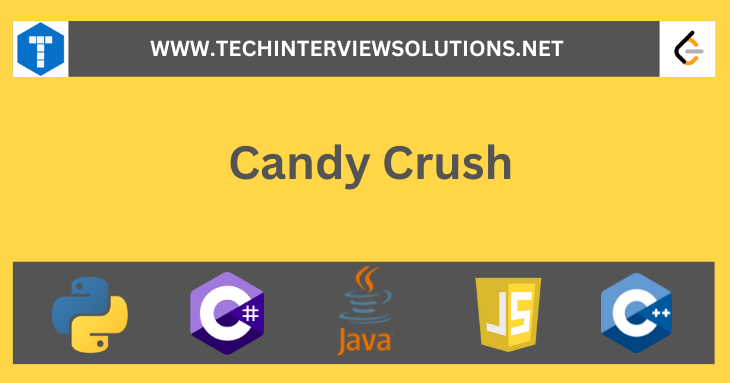
Candy Crush
This question is about implementing a basic elimination algorithm for Candy Crush.
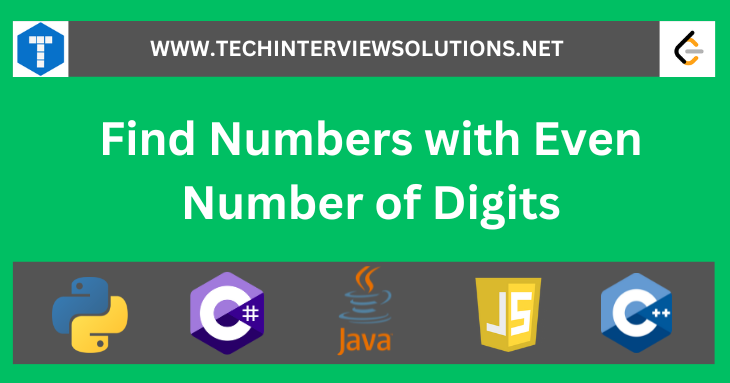
Find Numbers with Even Number of Digits
Given an array nums of integers, return how many of them contain an even number of digits.
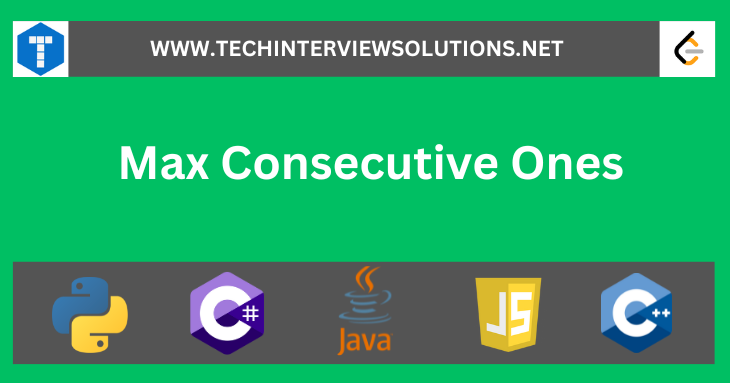
Max Consecutive Ones
Given a binary array nums, return the maximum number of consecutive 1's in the array.
In "LinkedList"
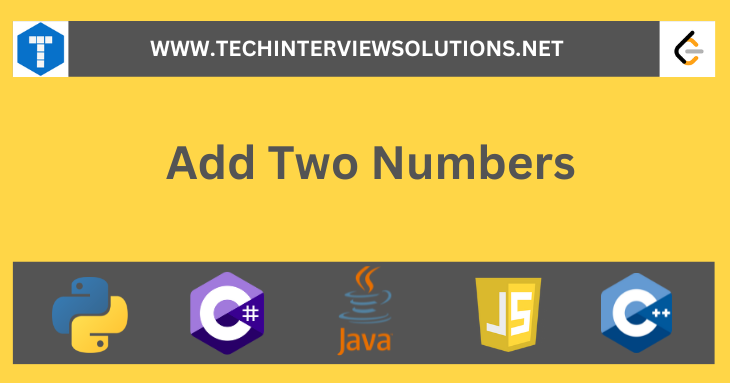
Add Two Numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order,...
In "Maths"
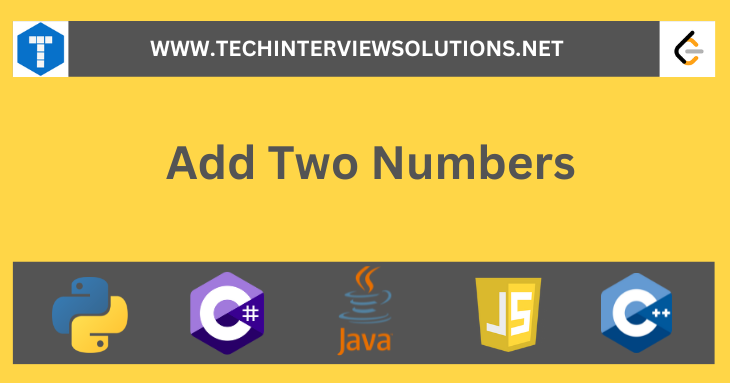
Add Two Numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order,...
In "Recursion"
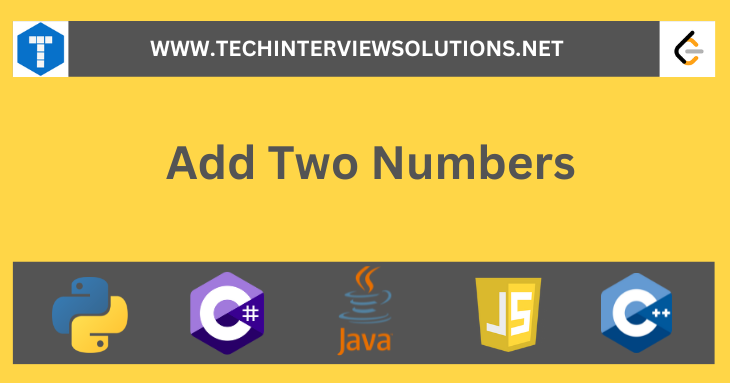
Add Two Numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order,...
In "TwoPointers"
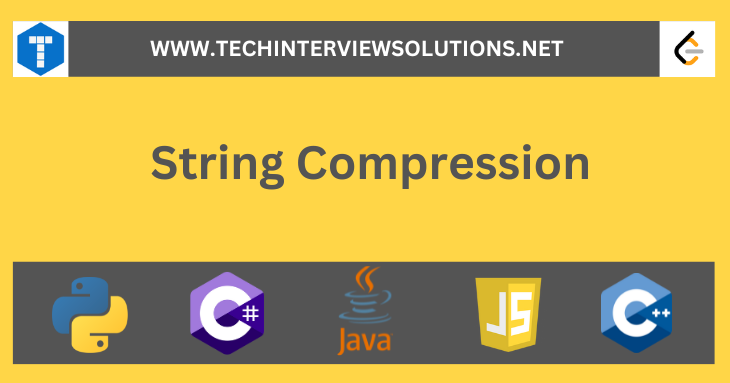
String Compression
Given an array of characters chars, compress it using the following algorithm:
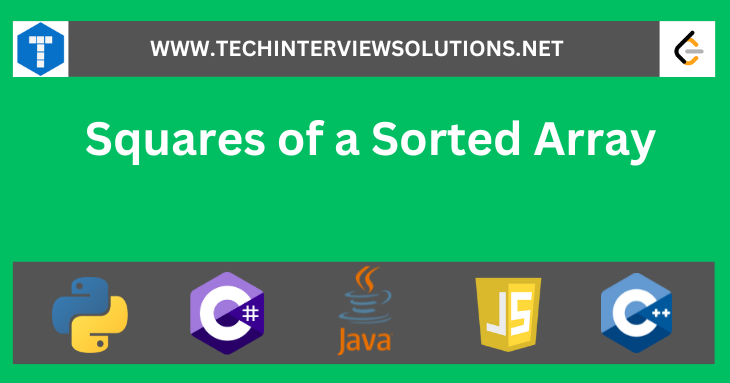
Squares of a Sorted Array
Given an integer array nums sorted in non-decreasing order, return an array of the squares of each number sorted in non-decreasing order....
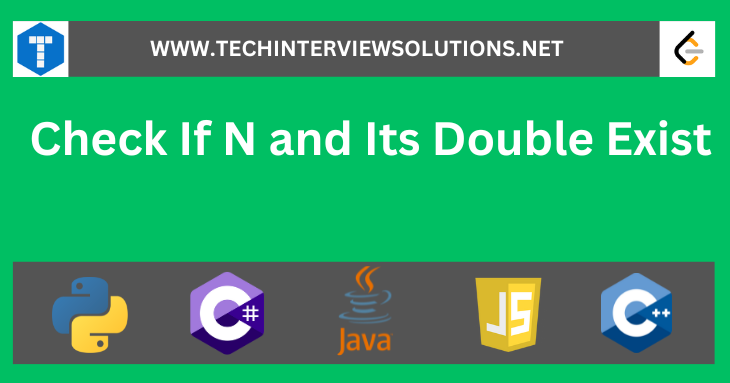
Check If N and Its Double Exist
Given an array arr of integers, check if there exist two indices i and j such that :
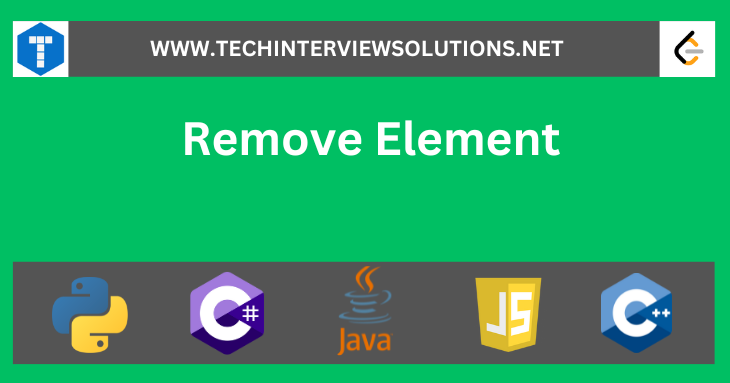
Remove Element
Given an integer array nums and an integer val, remove all occurrences of val in nums in-place. The relative order of the...
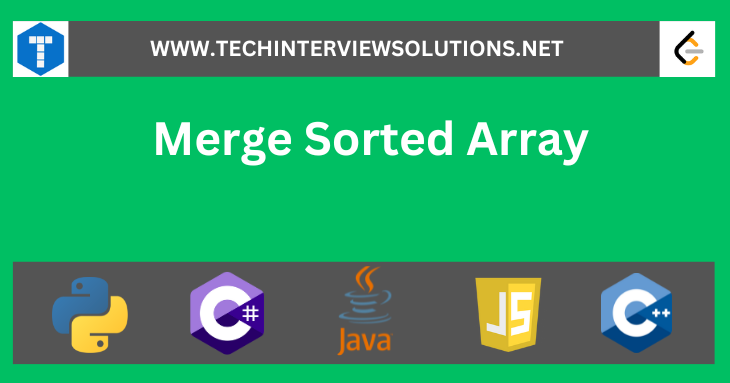
Merge Sorted Array
You are given two integer arrays nums1 and nums2, sorted in non-decreasing order, and two integers m and n, representing the number...
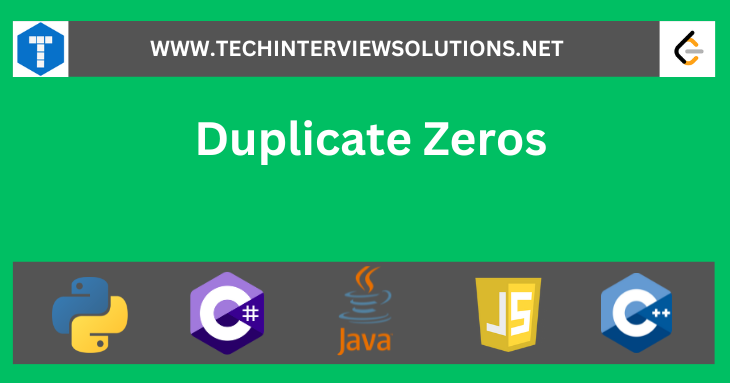
Duplicate Zeros
Given a fixed-length integer array arr, duplicate each occurrence of zero, shifting the remaining elements to...
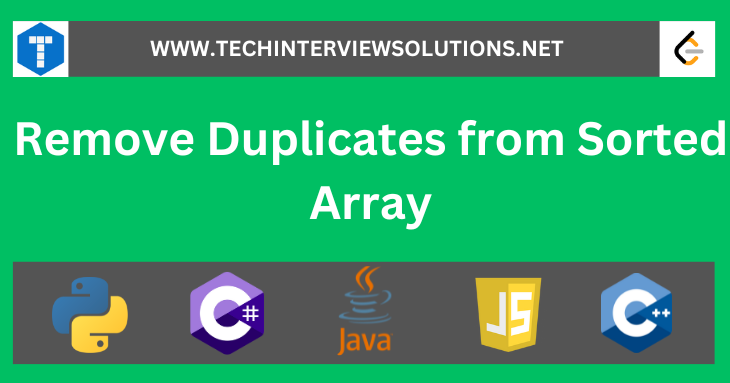
Remove Duplicates from Sorted Array
Given an integer array nums sorted in non-decreasing order, remove the duplicates in-place such that each unique element appears only once....
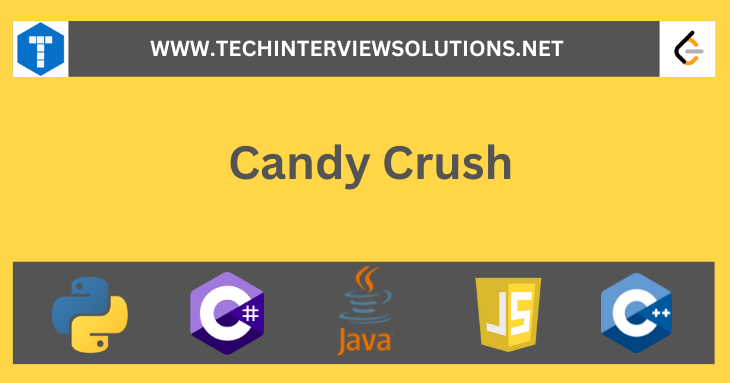
Candy Crush
This question is about implementing a basic elimination algorithm for Candy Crush.
In "Matrix"
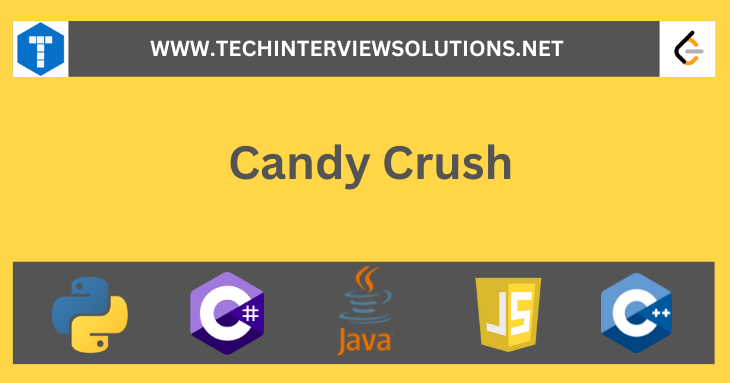
Candy Crush
This question is about implementing a basic elimination algorithm for Candy Crush.
In "Simulation"
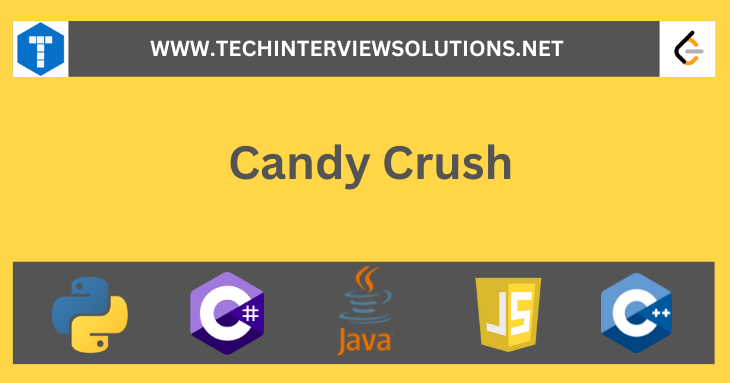
Candy Crush
This question is about implementing a basic elimination algorithm for Candy Crush.
In "HashTable"
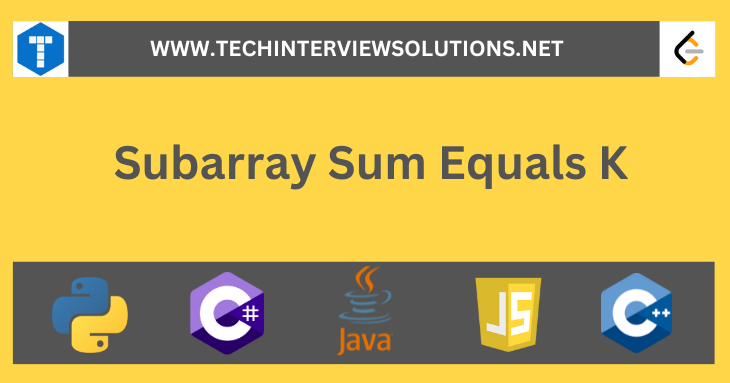
Subarray Sum Equals K
Given an array of integers nums and an integer k, return the total number of subarrays whose sum equals...
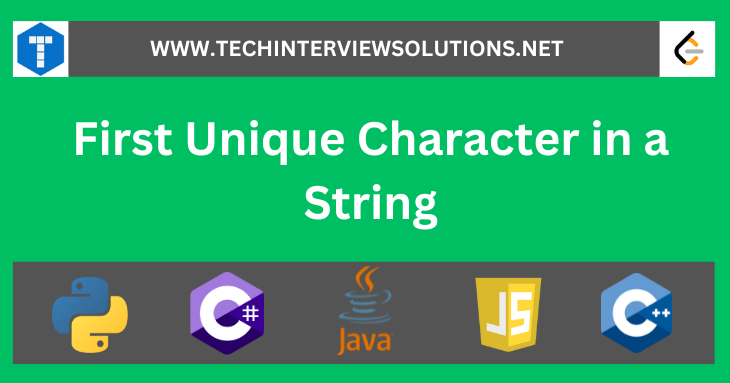
First Unique Character in a String
Given a string s, find the first non-repeating character in it and return its index ....
In "String"
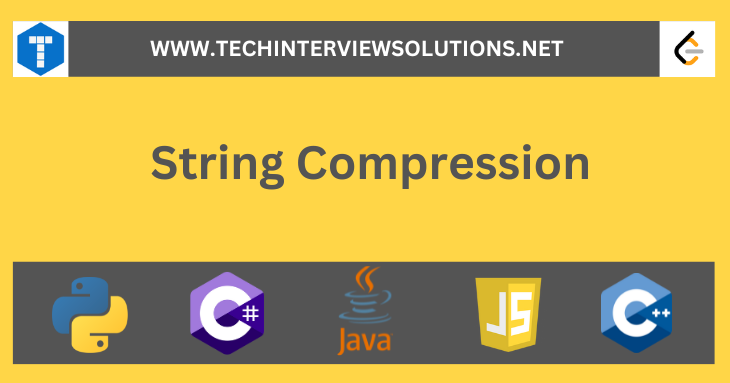
String Compression
Given an array of characters chars, compress it using the following algorithm:
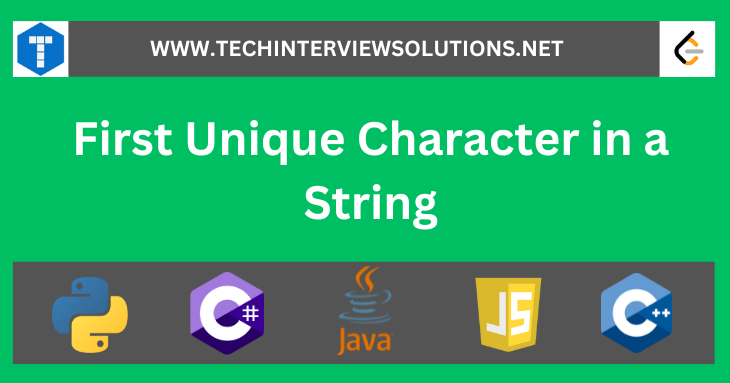
First Unique Character in a String
Given a string s, find the first non-repeating character in it and return its index ....
In "Queue"
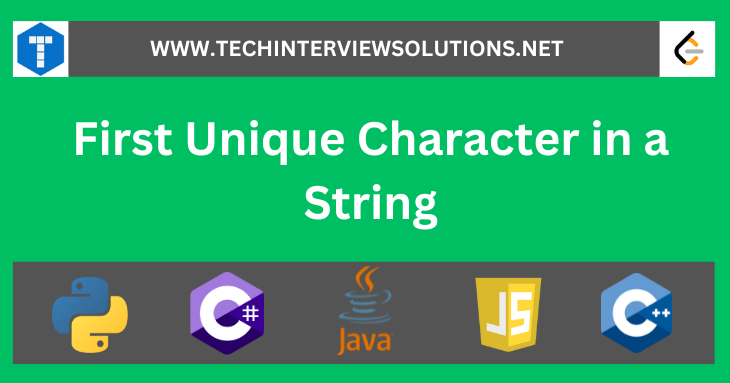
First Unique Character in a String
Given a string s, find the first non-repeating character in it and return its index ....
In "Counting"
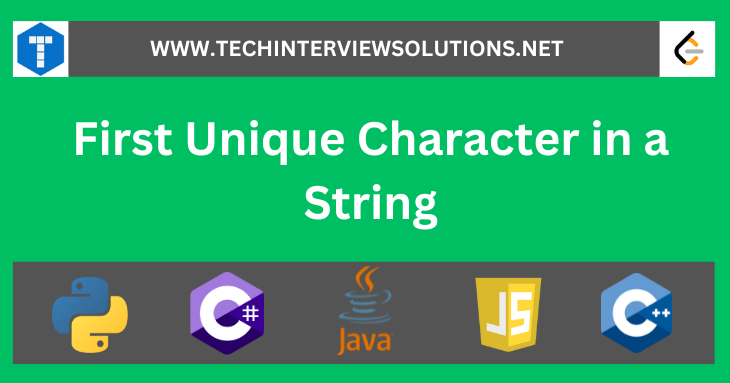
First Unique Character in a String
Given a string s, find the first non-repeating character in it and return its index ....
In "Sorting"
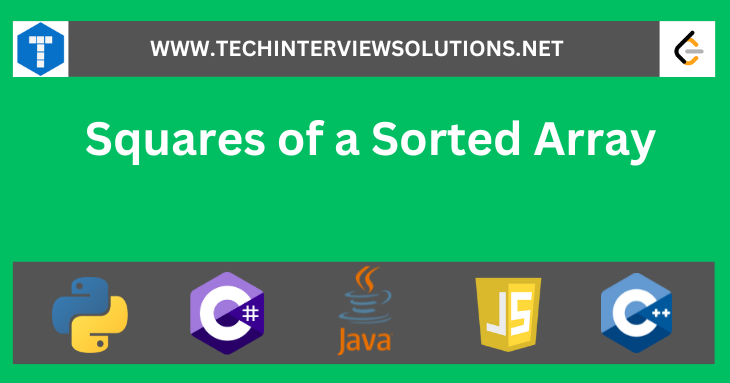
Squares of a Sorted Array
Given an integer array nums sorted in non-decreasing order, return an array of the squares of each number sorted in non-decreasing order....
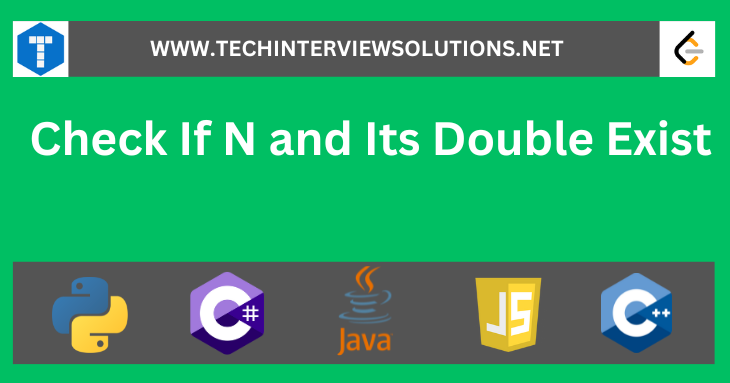
Check If N and Its Double Exist
Given an array arr of integers, check if there exist two indices i and j such that :
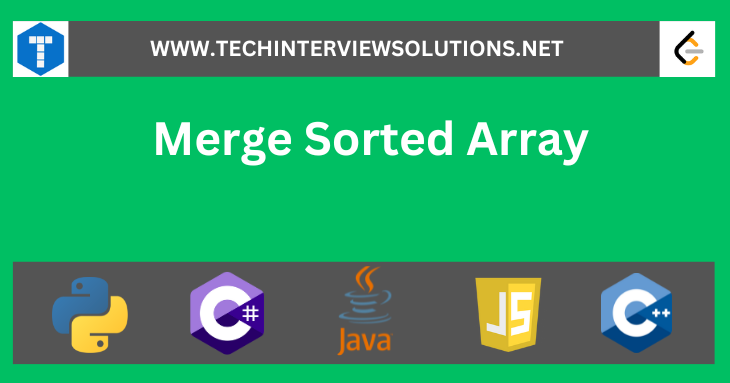
Merge Sorted Array
You are given two integer arrays nums1 and nums2, sorted in non-decreasing order, and two integers m and n, representing the number...
In "Hashing"
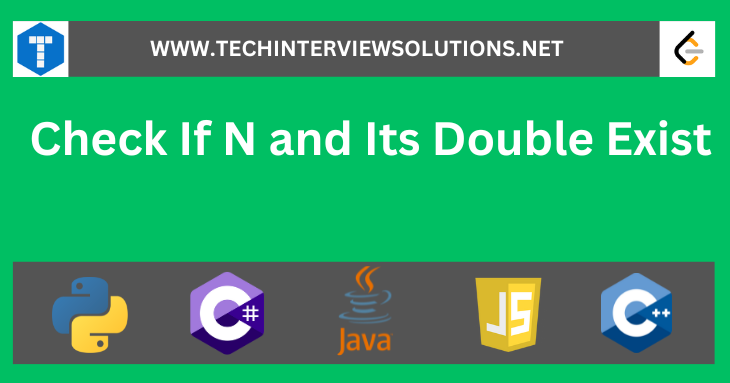
Check If N and Its Double Exist
Given an array arr of integers, check if there exist two indices i and j such that :
In "PrefixSum"
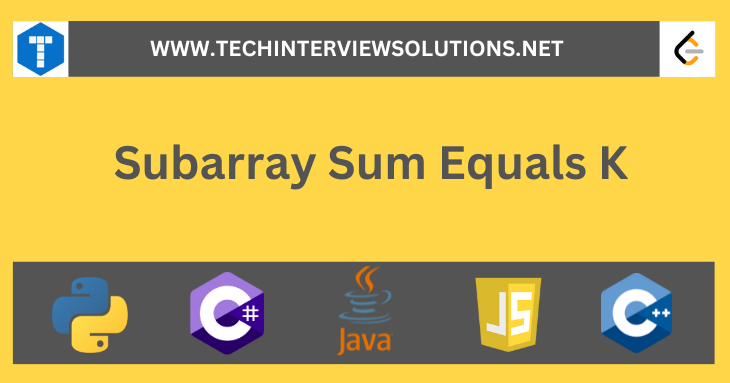
Subarray Sum Equals K
Given an array of integers nums and an integer k, return the total number of subarrays whose sum equals...
In "Tree"
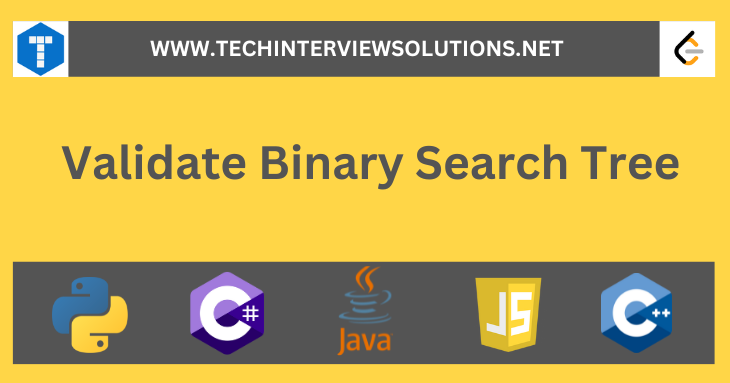
Validate Binary Search Tree
Given the root of a binary tree, determine if it is a valid binary search tree (BST).
In "BinaryTree"
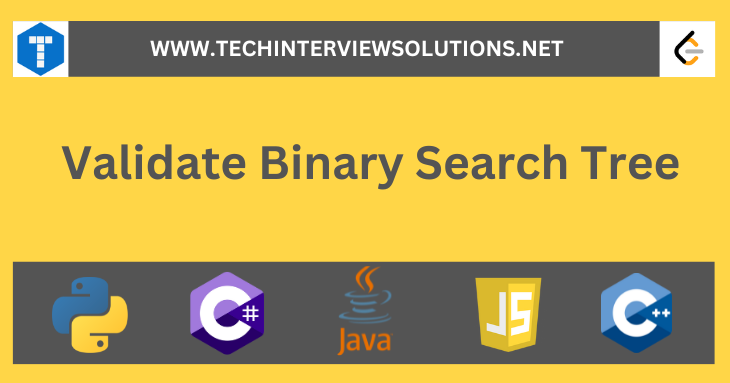
Validate Binary Search Tree
Given the root of a binary tree, determine if it is a valid binary search tree (BST).
In "BinarySearchTree"
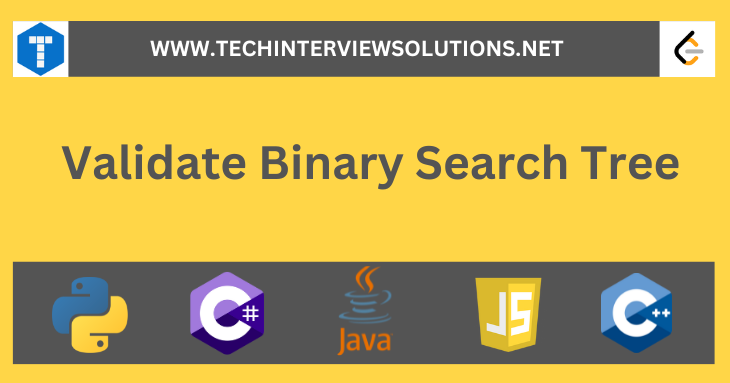
Validate Binary Search Tree
Given the root of a binary tree, determine if it is a valid binary search tree (BST).